Gun Assets
The ItemGunAsset class is used for ranged weapons (or “guns”), which can be used by players to deal damage. Some examples of vanilla ranged weapons include the Eaglefire and Crossbow.
Unity Asset Bundle Contents
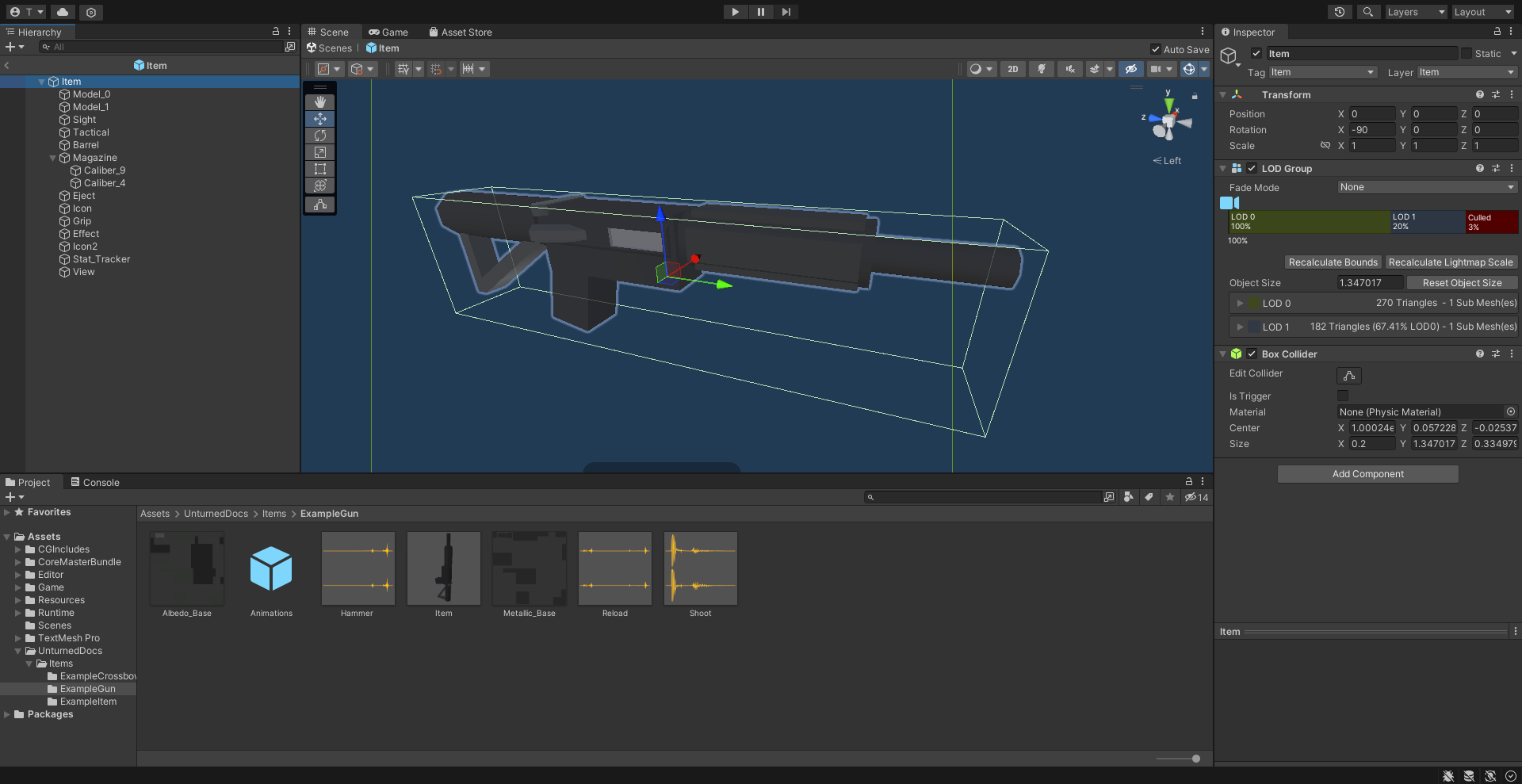
An example of a gun being set up in the Unity editor.
To get started, either follow the steps to begin creating a custom item from the introduction, or duplicate the contents of a prepackaged example asset.
Item (Prefab)
Open the “Item” Prefab, and add six child GameObjects named “Barrel”, “Grip”, “Sight”, “Tactical”, “Magazine”, and “Eject”. Most custom guns will want to have these six child GameObjects, although they are not strictly required.
The “Barrel”, “Grip”, “Sight”, “Tactical”, and “Magazine” GameObjects will determine the location of attachments on your gun. The “Sight” GameObject also determines where the camera will be positioned when aiming down sights. Shells are emitted from the “Eject” GameObject.
If an “View” GameObject is added, the camera will use its position when aiming down sights if a sight attachment has not been attached to the gun.
When a gun can accept more than one type of magazine caliber, it may be desirable to have the position of the magazine attachment depend on its caliber ID. Add a child to the “Magazine” GameObject, named “Caliber_#”. For example, adding “Caliber_1” would cause magazine attachments using caliber ID 1 to use that position instead of the “Magazine” GameObject’s position.
Additional Setup for Bows
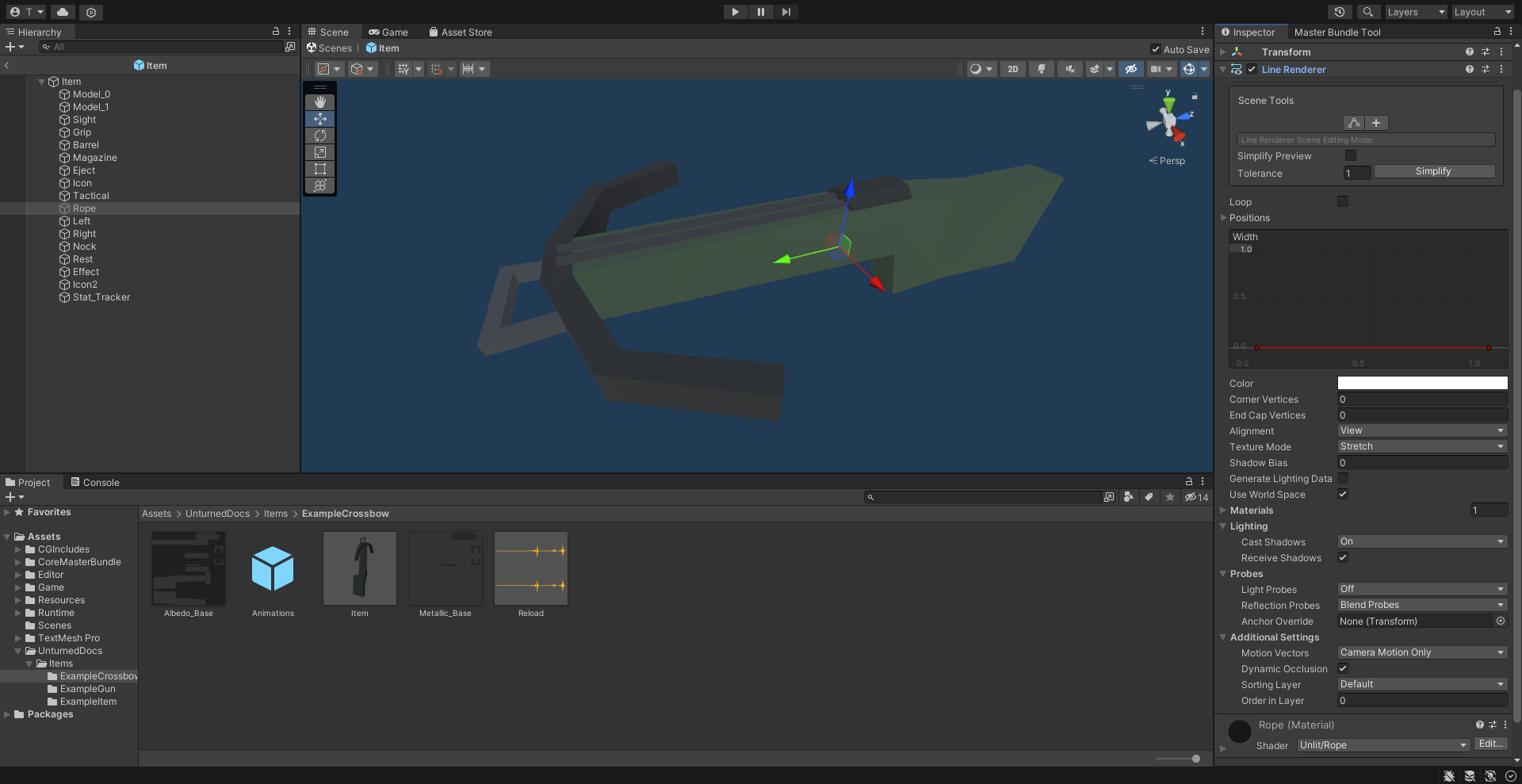
An example of a crossbow being set up in the Unity editor.
Bows require additional GameObjects to simulate the drawing of the bowstring. Note that bowstrings are only simulated from the first-person perspective.
Add a new child GameObject named “Rope”, and set its state to inactive. The “Rope” GameObject should include a Line Renderer component. Vanilla bowstrings use a custom Material named “Rope” with the Unlit-Rope Shader, but this is not required.
Add two child GameObjects named “Left” and “Right”. These GameObjects will determine the end points of the bowstring. If a third GameObject named “Rest” is included, it will be used as the middle point of the bowstring when aiming down sights.
Including a fourth GameObject named “Nock” will allow the bow to be fired without aiming down sights. Additionally, the “Rest” GameObject will act as a middle point when not aiming down sights, and the “Nock” GameObject will act as a middle point while aiming down sights.
Additional Setup for Economy Items
There are several child GameObjects that can be added related to skins. Custom items are ineligible to receive skins, so there is usually no reason to add these to the Prefab.
If an item has an “Icon2” GameObject included, its position and orientation will be used when generating icons of skins on this item. A GameObject named “Stat_Tracker” determines the location where stat trackers will appear on the gun, while a GameObject named “Effect” will determine the position of mythical effects on the gun.
Animations (Prefab)
In addition to animations used by any equippable item, guns have an additional set of animations that they can use.
Adding animations named “Aim_Start” and “Aim_Stop” will cause an animation to be played whenever the player starts or stops aiming down sights. Animations named “Attach_Start” and “Attach_Stop” will play when an attachment is attached or unattached to the gun. The “Sprint_Start” and “Sprint_Stop” animations play when the player starts and stops sprinting. The “Reload” animation is played when reloading the gun.
The “Hammer” animation is played under certain conditions where it would make sense to manually eject a cartridge from the gun. For example: after reloading an gun that had an empty magazine, or after firing a single-shot weapon (such as a bolt-action rifle or pump-action shotgun).
If a gun is configured to use the gun jamming feature, the “UnjamChamber” animation will play when a jam occurs.
Audio Clips
In addition to the Audio Clips that can be included for equippable items, guns have an additional set of audio clips they can use.
If an Audio Clip named “Shoot” is included, it will play after the gun is fired. Including Audio Clips named “Reload” and “Hammer” will cause audio to play after reloading and hammering the gun, respectively.
An “Aim” Audio Clip can be included to have audio play after aiming down sights. For example, a longbow might want to have an the sound of the bow being drawn play. Miniguns can also include an Audio Clip named “Minigun” to have audio play while revving the minigun.
If a gun is configured to use the gun jamming feature, the “ChamberJammed” Audio Clip will play when a jam occurs.
Game Data File
Ranged weapons inherit properties from the ItemWeaponAsset class. Any properties from parent classes that are required—or highly recommended—are listed in the table below.
Class |
Property Name |
Required Value |
---|---|---|
|
||
|
||
Additionally, all ranged weapons require that the Action
property has been configured. Note that ranged weapons will always show a quality value.
Properties
Ranged weapons have a significant number of properties. To make navigating these easier, they have been categorized into one of several property tables. Many of these tables contain similar properties that are often used together.
Property Name |
Type |
Default Value |
---|---|---|
|
||
See description |
||
|
||
|
||
See description |
||
See description |
||
|
Property Name |
Type |
Default Value |
---|---|---|
See description |
||
See description |
||
|
||
See description |
||
See description |
||
|
Property Name |
Type |
Default Value |
---|---|---|
|
||
|
||
|
||
|
Property Name |
Type |
Default Value |
---|---|---|
|
||
|
||
See description |
Property Name |
Type |
Default Value |
---|---|---|
|
||
|
||
|
||
Property Name |
Type |
Default Value |
---|---|---|
|
||
|
||
|
||
|
||
Property Name |
Type |
Default Value |
---|---|---|
|
||
|
||
|
Property Name |
Type |
Default Value |
---|---|---|
|
||
|
||
|
||
|
||
deprecated |
||
|
||
|
||
|
||
|
||
|
||
|
||
|
||
See description |
||
|
Property Name |
Type |
Default Value |
---|---|---|
deprecated |
||
See description |
||
See description |
||
|
Property Name |
Type |
Default Value |
---|---|---|
|
||
See description |
||
|
||
Property Name |
Type |
Default Value |
---|---|---|
|
||
|
||
|
||
|
||
|
||
|
||
|
||
|
||
|
||
|
Property Name |
Type |
Default Value |
---|---|---|
|
||
|
||
|
||
|
||
|
||
|
Property Name |
Type |
Default Value |
---|---|---|
|
||
|
||
|
||
deprecated |
||
|
||
|
EAction Enumeration
Named Value |
Description |
---|---|
|
Corresponds to the “Trigger” action. Uses the ballistic projectile system. |
|
Corresponds to the “Bolt” action. Uses the ballistic projectile system. |
|
Corresponds to the “Pump” action. Uses the ballistic projectile system. |
|
Corresponds to the “Rail” action. Uses the ballistic projectile system. |
|
Corresponds to the “String” action. Uses the ballistic projectile system. |
|
Corresponds to the “Break” action. Uses the ballistic projectile system. |
|
Corresponds to the “Rocket” action. Uses the physics projectile system. |
|
Corresponds to the “Minigun” action. Uses the ballistic projectile system. |
Property Descriptions
Action EAction
The value of this property determines how the weapon functions when used, including whether it uses ballistic projectiles, or physics projectiles. Different properties are available to the weapon depending on the value of this property.
Although most action mechanisms utilize ballistic projectiles, the Rocket
action mechanism uses physics projectiles instead. Additionally, any projectiles from these weapons (e.g., the Rocket Launcher) are explosive.
To fire a weapon with the String
action mechanism, a player must be aiming down sights – unless a “Nock” GameObject has been added during its Unity setup.
Aim_In_Duration float32 0.2
How long it takes to fully aim down sights, in seconds.
Aiming_Movement_Speed_Multiplier float32
Multiplier on the player’s movement speed while aiming down sights. Defaults to 0.75
when Can_Aim_During_Sprint
is false
. Otherwise, defaults to 1
.
Aiming_Recoil_Multiplier float32 1
Multiplier on recoil magnitude while aiming down sights.
Alert_Radius float32 48
The radius of the alert generated by ranged weapons when they are fired. Zombies or animals caught within this radius are alerted. This radius is measured in meters.
Allow_Magazine_Change bool true
When false
, the magazine cannot be removed, replaced, or reloaded. This functions similar to a few other properties, such as Hook_Barrel
or Hook_Grip
when determing valid hook attachment slots.
Ammo_Max uint8 0
Maximum amount of ammo to randomly generate in the magazine attachment that was attached to the weapon by default.
Ammo_Min uint8 0
Minimum amount of ammo to randomly generate in the magazine attachment that was attached to the weapon by default.
Ammo_Per_Shot uint8 1
Number of ammunition consumed per shot. For example, a value of 3
would consume three ammo every time the weapon is fired, while a value of 0
would allow for the weapon to have infinite ammo.
Attachment_Caliber_# uint16
Legacy ID of a caliber to check for hook attachment compatibility. This property is used in conjunction with Attachment_Calibers
, which determines how many instances of this property should be read by the game.
When this property is unset, it will default to 0
. When the Attachment_Calibers
property is not greater than 0
, this property will default to the value of any Magazine_Caliber_#
properties.
For example, a valid configuration for a ranged weapon’s calibers could be:
Attachment_Calibers 2
Attachment_Caliber_0 1
Attachment_Caliber_1 9
Magazine_Calibers 3
Magazine_Caliber_0 1
Magazine_Caliber_1 4
Magazine_Caliber_2 9
This would allow the ranged weapon to use hook attachments with caliber IDs of 1 or 9, and to use magazine attachments with caliber IDs of 1, 4, or 9.
Attachment_Calibers int32
Set the length of the array containing the calibers for hook attachment compatibility. This property is used in conjunction with the Attachment_Caliber_#
property, and the value of Attachment_Calibers
should be equal to the number of instances of Attachment_Caliber_#
.
When this property is not greater than 0
– it will default to the value of Magazine_Calibers
, and the Attachment_Caliber_#
property can no longer be customized.
To use this property, Magazine_Calibers
must be configured.
Auto flag
The weapon has an automatic firing mode.
Ballistic_Drop float32
Deprecated since version 3.23.7.0: Use Bullet_Gravity_Multiplier
instead.
Existing values are automatically converted if Bullet_Gravity_Multiplier
has not been configured. The conversion is logged during Asset Validation.
Ballistic_Force float32 0.002
The amount of force that should be applied to the physics projectile, measured in Newtons. It may be helpful to read Unity’s Rigidbody.AddForce documentation to better understand physics projectiles.
Properties used by physics projectiles (such as Ballistic_Force
) cannot be used alongside properties intended for ballistic projectiles (such as Ballistic_Travel
or Bullet_Gravity_Multiplier
).
Ballistic_Steps uint8
Lifespan of ballistic projectiles. A higher value relative to Ballistic_Travel
will result in less muzzle velocity. Must be a value greater than 0
.
Defaults to Range ÷ Ballistic_Travel
, rounded up to the nearest integer.
To avoid a mismatch between the weapon’s max range and its manual ballistic range, it is recommend to only configure Ballistic_Steps
or Ballistic_Travel
(or neither) – no both.
Ballistic_Travel float32
Travel speed of ballistic projectiles. A higher value relative to Ballistic_Steps
will result in more muzzle velocity. Must be a value greater than 0.1
.
Defaults to 10
. If Ballistic_Steps
is specified and greater than 0
, and Ballistic_Travel
is not specified, then Ballistic_Travel
defaults to Range ÷ Ballistic_Steps
.
To avoid a mismatch between the weapon’s max range and its manual ballistic range, it is recommend to only configure Ballistic_Travel
or Ballistic_Steps
(or neither) – no both.
Barrel uint16 0
Legacy ID of a barrel attachment that should be attached by default. The Hook_Barrel
flag is not required to use this property.
Bullet_Gravity_Multiplier float32 4
Multiplier for gravity’s acceleration. This property is available to ballistic projectile weapons. Setting this value to 1
allows for more realistic bullet drop.
Note
This defaults to 4
because Unturned’s maximum engagement distance is rather short, but this distance may be raised in the future if/when network improvements are made to the game. Gravity defaults to 9.81 m/s², or can be configured in the Level Config.
Bursts int32 0
When a value greater than 0
is provided, the weapon has a burst firing mode. A number of shots equal to this value is fired when using this mode.
Caliber uint16 0
Legacy ID of the caliber to check for hook attachment and magazine attachment compatibility. To add compatibility for multiple calibers, or to configure hook attachment and magazine attachment compatibility separately, use the Magazine_Calibers
and Attachment_Calibers
properties instead.
Can_Aim_During_Sprint bool false
When true
, the player can sprint while aiming down sights.
Can_Ever_Jam flag
When this flag is included, the weapon can jam. Weapons have a chance of jamming once their quality drops below a certain threshold. Starting from the initial threshold, the chance of jamming on each shot is blended between between 0% and a specified max chance.
The “ChamberJammed” Audio Clip is played when a jam occurs, as well as the animation “UnjamChamber” if present.
For an example, refer to .../Guns/Cobra_Jam/Cobra_Jam.dat
in the game files.
Damage_Falloff_Max_Range float32 1
Percentage of maximum range where damage stops decreasing. For example, a max falloff range value of 0.6
with a range of 200
means damage stops dropping off after 120 meters.
Damage_Falloff_Multiplier float32 1
Percentage of damage to apply at maximum range. For example, a falloff multiplier value of 0.25
with a damage value of 40
means 10 damage will be dealt at maximum range.
Damage_Falloff_Range float32 1
Percentage of maximum range where damage begins decreasing. For example, a falloff range value of 0.3
with a range value of 200
means damage begins dropping off after 60 meters.
Delete_Empty_Magazines flag
Deprecated since version 3.30.3.0: Use Should_Delete_Empty_Magazines
instead.
When this flag is included, the attached magazine attachment is deleted when fully depleted.
Explosion GUID or uint16
GUID or legacy ID of the effect that should be used for explosions caused by Action Rocket
projectiles.
Fire_Delay_Seconds int32 0
Delay before the weapon is actually fired, in seconds.
Firerate uint8 0
The value of this property affects the minimum number of ticks between the firing of consecutive shots. A higher Firerate
value will cause the weapon to have a slower rate of a fire. The weapon’s rate of fire can be calculated with 50 ÷ (Firerate + 1)
, as the rounds per second.
Grip uint16 0
Legacy ID of a grip attachment that should be attached by default. The Hook_Grip
flag is not required to use this property.
Gunshot_Rolloff_Distance float32
Distance over which the gunshot audio rolls off until it is completely inaudible, in meters. Defaults to 16
when using Action String
; defaults to 64
when using Action Rocket
; otherwise, defaults to 512
.
Hammer_Time float32 1
Multiplier on the time it takes to pull back the hammer a ranged weapon after firing. This does not affect the actual animation speed, but the cooldown before the player can perform other actions (such as shooting) again. Values less than 1
have no effect.
Hook_Barrel flag
When this flag is included, the ranged weapon has a barrel attachment slot.
Hook_Grip flag
When this flag is included, the ranged weapon has a grip attachment slot.
Hook_Sight flag
When this flag is included, the ranged weapon has a sight attachment slot.
Hook_Tactical flag
When this flag is included, the ranged weapon has a tactical attachment slot.
Infinite_Ammo bool false
When true
, ammunition is not depleted from the magazine attachment. This allows for the weapon to have infinite ammo, so long as a magazine attachment with a number of rounds remaining equal to Ammo_Per_Shot
is attached.
Instakill_Headshots bool false
If true
, a player that is headshot with this weapon is instantly killed. This does not affect zombies, unless the world’s difficulty configuration has the Weapons_Use_Player_Damage
setting enabled.
Jam_Max_Chance float32 0.1
Decimal-to-percent chance for jamming to occur. This property requires Can_Ever_Jam
.
Jam_Quality_Threshold float32 0.4
The maximum threshold for when jamming can occur. This value is a decimal-to-percent representation of the item’s quality value. For example, a threshold of 0.4
allows jamming to start occuring at 40% item quality. This property requires Can_Ever_Jam
.
Magazine uint16 0
Legacy ID of a magazine attachment that should be attached by default.
Magazine_Caliber_# uint16
Legacy ID of a caliber to check for magazine attachment compatibility. This property is used in conjunction with Magazine_Calibers
, which determines how many instances of this property should be read by the game.
When this property is unset, it will default to 0
. When the Magazine_Calibers
property is not greater than 0
, this property will default to the value of Caliber
.
Magazine_Calibers int32
Set the length of the array containing the calibers for magazine attachment compatibility. This property is used in conjunction with the Magazine_Caliber_#
property, and the value of Magazine_Calibers
should be equal to the number of instances of Magazine_Caliber_#
.
When this property is not greater than 0
– it will default to 1
, and the Magazine_Caliber_#
property can no longer be customized.
This property is often used alongside Attachment_Calibers
, but this is optional.
Magazine_Replacement_#_ID uint16 0
Legacy ID of a magazine attachment that should be used as an alternative default when certain condition(s) are met. This property is used in conjunction with Magazine_Replacements
, which determines how many instances of this property should be read by the game.
Magazine_Replacement_#_Map string
This value should be the name of a map. When the weapon spawns on this map, this condition has been met. This property requires Magazine_Replacement_#_ID
.
Magazine_Replacements int 0
Magazine_Replacements
and its related properties are used to add alternative magazine attachments that should be used as the weapon’s default when certain condition(s) are met.
This value sets the length of the array containing any alternative default magazine attachments. This property is used in conjunction with the Magazine_Replacement_#_ID
property, and the value of Magazine_Replacements
should be equal to the number of instances of Magazine_Replacement_#_ID
.
Muzzle GUID or uint16
GUID or legacy ID of the effect to play after shooting. This is emitted from the ranged weapon’s “Barrel” GameObject.
Projectile_Explosion_Launch_Speed float32
Players caught within the area-of-effect explosion caused by a physics projectile weapon are launched at this speed. For example, this can be used to create velocity-related items like “rocket-jumping” mods. Defaults to Player_Damage × 0.1
.
Projectile_Lifespan float32 30
Lifespan of physics projectiles, in seconds. After this much time elapses, the projectile despawns.
Projectile_Penetrate_Buildables flag
The area-of-effect explosions caused by physics projectiles penetrate through buildables when this flag is set.
Range_Rangefinder float32
Overrides the maximum distance displayed when using a “Rangefinder” tactical attachment on this weapon. For example, it may be useful to set this property when using Action Rocket
, as explosive projectiles use Range
to determine the explosion radius rather than the maximum range of the weapon. Defaults to the value of the Range
property.
Recoil_Crouch float32 0.85
Multiplier on camera recoil while crouched.
Recoil_Max_X float32 0
Maximum horizontal camera recoil in degrees. This property is used in conjunction with Recoil_Min_Y
.
Recoil_Max_Y float32 0
Maximum vertical camera recoil in degrees. This property is used in conjunction with Recoil_Min_X
.
Recoil_Min_X float32 0
Minimum horizontal camera recoil in degrees. This property is used in conjunction with Recoil_Max_X
.
Recoil_Min_Y float32 0
Minimum vertical camera recoil in degrees. This property is used in conjunction with Recoil_Max_Y
.
Recoil_Prone float32 0.7
Multiplier on camera recoil while prone.
Recoil_Sprint float32 1.25
Multiplier on camera recoil while sprinting. This property is not relevant unless Can_Aim_During_Sprint
has been set to true
.
Recover_X float32 0
Multiplier on camera degrees to be counter-animated horizontally over the next 250 milliseconds.
Recover_Y float32 0
Multiplier on camera degrees to be counter-animated vertically over the next 250 milliseconds.
Reload_Time float32 1
Multiplier on time it takes to finish reloading the ranged weapon. This does not affect the actual animation speed, but the cooldown before the player can perform other actions (such as shooting) again. Values less than 1
have no effect.
Replace float32 1
Multiplier of the reload animation length before the magazine is respawned. This does not affect the actual animation speed, but the cooldown before the player can perform other actions (such as shooting) again. Values less than 0.01
have no effect.
Requires_NonZero_Attachment_Caliber bool false
If true
, attachments must specify at least one non-zero (0
) caliber ID to be compatible. For example, this can be used to make most vanilla attachments (like the Tactical Laser, Dot Sight, and Vertical Grip) incompatible with this weapon.
Safety flag
The weapon has a safety firing mode.
Scale_Aim_Animation_Speed bool true
When true, the length of the “Aim_Start” and “Aim_Stop” animations are scaled to match Aim_In_Duration
(with modifiers).
Semi flag
The weapon has a semi-automatic firing mode.
Shake_Max_X float32 0
Maximum 𝘟-axis model shake caused from firing the weapon.
Shake_Min_X float32 0
Minimum 𝘟-axis model shake caused from firing the weapon.
Shake_Max_Y float32 0
Maximum 𝘠-axis model shake caused from firing the weapon.
Shake_Min_Y float32 0
Minimum 𝘠-axis model shake caused from firing the weapon.
Shake_Max_Z float32 0
Maximum 𝘡-axis model shake caused from firing the weapon.
Shake_Min_Z float32 0
Minimum 𝘡-axis model shake caused from firing the weapon.
Shell GUID or uint16
GUID or legacy ID of the effect to play after shooting, emitted from the ranged weapon’s “Eject” GameObject. Defaults to 33
when using either Action Pump
or Action Break
; defaults to 1
when using any other Action
key-value pair except for Action Rail
; otherwise, defaults to 0
.
Should_Delete_Empty_Magazines bool
Overrides how empty magazines are handled by the action item mode. When set to true
, empty magazine attachments are deleted when completely emptied. The default behavior depends on the configuration of the Action
property.
Defaults to true
when using one of the following Action
enumerators: Break
, Pump
, Rail
, Rocket
, or String
. Otherwise, defaults to false
.
Sight uint16 0
Legacy ID of a sight attachment that should be attached by default. The Hook_Sight
flag is not required to use this property.
Spread_Aim float32 0
Multiplier on the bullet spread while aiming down sights. This is multiplied by the Spread_Angle_Degrees
value.
Spread_Angle_Degrees float32 0
Bullet angle of deviation away from the aiming direction. For example, 15
means the shot could hit up to 15 degrees away from the center of the crosshair, whereas 0
will always hit the center of the crosshair. All other spread values are multipliers for this.
Spread_Crouch float32 0.85
Multiplier on the bullet spread while crouched.
Spread_Hip float32
Deprecated since version 3.22.20.0: Use Spread_Angle_Degrees
instead.
Maintained for backwards compatibility. Running the game with the -ValidateAssets
launch option will log the equivalent Spread_Angle_Degrees
value.
Spread_Prone float32 0.7
Multiplier on the bullet spread while prone.
Spread_Sprint float32 1.25
Multiplier on the bullet spread while sprinting.
Tactical uint16 0
Legacy ID of a tactical attachment that should be attached by default. The Hook_Tactical
flag is not required to use this property.
Turret flag
This weapon should be treated as a vehicular turret. This flag affects the player’s first-person viewmodel while the weapon is held.
Unjam_Chamber_Anim string UnjamChamber
Name of an animation clip to play when unjamming the weapon. This property requires Can_Ever_Jam
.
Unplace float32 0
Multiplier of the reload animation length before the magazine is despawned. This does not affect the actual animation speed, but the cooldown before the player can perform other actions (such as shooting) again.
NPC Rewards
Gun assets can use quest rewards. For example, every time the ranged weapon is fired an item could be spawned in the player’s inventory. Alternatively, shooting the ranged weapon may be required to complete a quest. For more information, refer to the Rewards documentation.
These rewards are prefixed with Shoot_Quest_
. For example, Shoot_Quest_Rewards 1
.
Understanding Projectile Systems
All ranged weapons utilize one of two projectile systems: the ballistic projectile system, or the physics projectile system. This is determined based on the Action the weapon has been configured to use, although most weapons use the ballistic projectile system.
Ballistic projectiles use a deterministic simulation. Their travel time, bullet drop, and other characteristics can be configured with properties such as Ballistic_Travel and Bullet_Gravity_Multiplier. When the ballistics game mechanic is disabled, these weapons function as hitscan instead.
Physics projectiles use Unity’s physics simulation. Unlike ballistic projectiles, these are not deterministic. Additionally, physics projectiles cause area-of-effect explosions upon impact. The characteristics of physics projectiles can be configured with properties such as Ballistic_Force and Projectile_Explosion_Launch_Speed.